Introduction to LINQ(For You Masochists Out There)
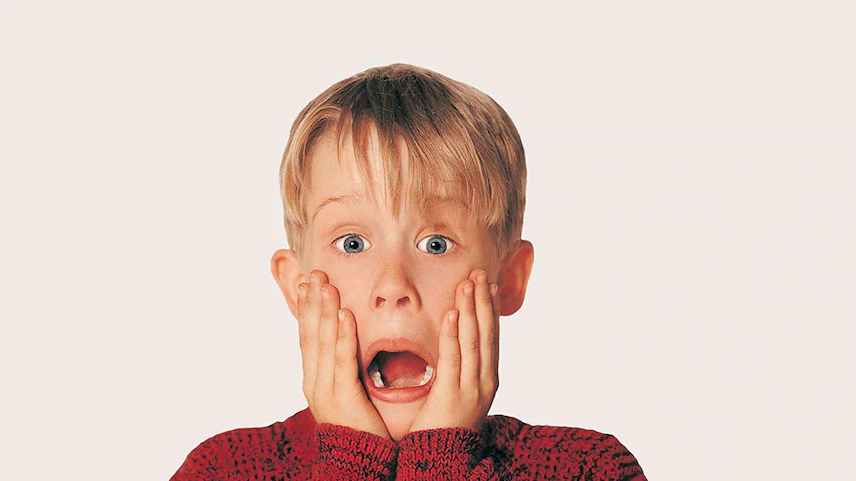
If you wish to skip past my rambling and head over into the actual introduction, click here.
Language Integrated Query is a pretty neat feature in .Net technology that helps us query data from various data sources with ease while also evoking the same reaction you see in the poster above, when people come across it for the first time.
But that definition is lacking in detail.
Let’s see what Google has to say about LINQ.
Language Integrated Query (LINQ, pronounced “link”) is a Microsoft . NET Framework component that adds native data querying capabilities to .NET languages
I see.
I must have accidentally switched the language to Japanese when I was busy searching for the latest One Piece comic.
I’ll switch it back to English and see what Sir Google has to say, now that we know what Google-san had to say about it.
LINQ is integrated in C# or VB, thereby eliminating the mismatch between programming languages and databases, as well as providing a single querying interface for different types of data sources.
Sir Google has done the impossible by explaining everything, and nothing at the same time.
Those who understand LINQ will understand this definition, while those who are trying to understand LINQ might have fallen asleep after reading this.
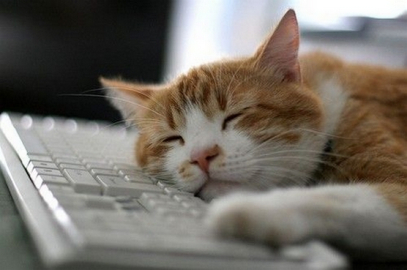
As you can see, I struggled to learn LINQ because Sir Google and Google-san have made it their life’s mission to put people off from learning advanced concepts in .Net programming.
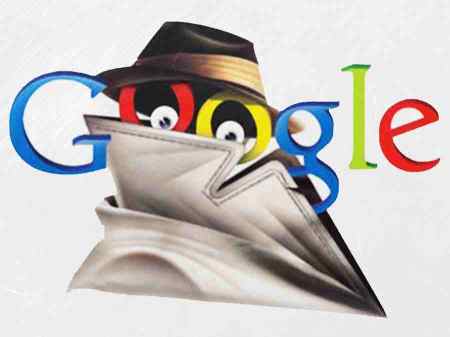
Leaving all that side, LINQ is pretty awesome.
It wasn’t until I started working with it, that I saw just how powerful it was.
But before we dive into developing LINQs, we have to understand what it is first.
What is LINQ?
Microsoft realized that retrieving data of different types and from various sources and formats, into particular formats wasn’t easy. Reading that last sentence itself wasn’t particularly easy either.
Our prehistoric developers were forced to learn new querying languages each time they had to interact with different data sources, so you can imagine the struggle they faced.
But Microsoft, being Microsoft figured something out, and it was ingenious.
That something was a common querying interface that would enable you to use the language you are currently using, to query data from not just one but multiple sources.
Now you just have to use your programming language like you usually do, without having to worry about the specifics, hence the name.
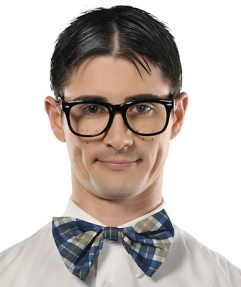
Shouldn’t It Be LIQ?
Microsoft wanted to emphasis its ability to Link data from any source, regardless of the type of data we were interested in retrieving, hence the pronunciation.
Linking data sounds better that Liqing data which sounds…wrong.
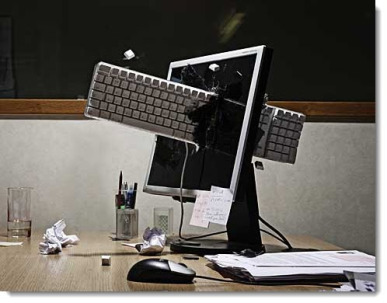
However, we won’t be covering LINQ in depth here, because I am selfish learning it myself. I just know how to use it in UiPath, and I will teach you whatever I have learned so far because I am desperate for your attention love sharing what I learn.
This is a blog dedicated to RPA, and we will use LINQ insofar as it benefits us as RPA developers.
UiPath and LINQ
UiPath automations can be written either in VB.Net or C#.
We tend to develop our codes using VB.Net since C# was released later on.
VB.Net is almost identical to C#, so you won’t have much trouble converting my VB.Net LINQs into C#.
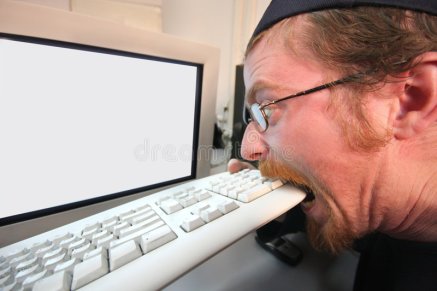
Try that as an exercise, it will benefit you in the long run.
Also, it keeps things interesting doesn’t it?
Teaser
Here is a sneak peak of what is to come.
LINQ can be developed either by using methods, or by using queries. Given below is an example of a Method Syntax LINQ.
ArrayOfIntegers.Sum
This bit of code retrieves the sum of all integers present in your array of integers. The .Sum appended to the end of our LINQ is something we call an extension method. They are ready made functions which are already present in our Studio, that we may avail at any time.
In order to use extension methods, we have to append them to the ends and in-betweens of our codes.
Methods are slightly easier to understand, as witnessed above, so we will stick to them for this example.
However, if you are comfortable with querying languages, then the following approach would be more to your liking:
(From i In ArrayOfIntegers
Select i).Sum
This might leave you confused, since we are using both query syntax, as well as an extension method to get our sum back.
But this does seem inefficient, since we are referencing more methods, right?
That is because this is a relatively simple problem.
When dealing with complex requirements, it is best to use query, or a mix of both to achieve the desired output.
But just for the sake of showing you what a simple query syntax would look like, say you were only interested in summing up values that are multiples of 2.
(From i In ArrayOfIntegers
Select If((i Mod 2 = 0), i, 0)).Sum
Querying allows us to separate our conditions into various segments and aliases(names for the local variables).
This make it readable and much easier to maintain. In the following example, Integers is used as an alias for the result we wish to retrieve.
(From i In ArrayOfIntegers
Let Integers = If((i Mod 2 = 0), i, 0)
Select Integers).Sum
That’s what makes it so darn useful.
But the right way to retrieve our result in this case would be to use Where like so:
(From i In ArrayOfIntegers
Where (i Mod 2 = 0)
Select Integers).Sum
Now, onto our next example.
Say you have an array consisting of integer values which contains duplicate, and you wish to extract unique values from them.
ArrayOfIntegers = {1,2,2,3,3,4,5,5,5,5,6}
How would you achieve that output using the click and drag activities you are familiar with in UiPath?
You’d probably drag in a For Each Activity, assign the Array there, loop through the entire set, provide appropriate conditions and collect unique items one by one.
That works Well so Why Bother Learning LINQ?
Ah! I was hoping you would ask.
Imagine your manager walks into the office, pushes you aside, hammers the keyboard like a monkey high on caffeine, adding 10000 items into your array, before heading back to the pantry to get himself another cup of caffeinated caffeine.
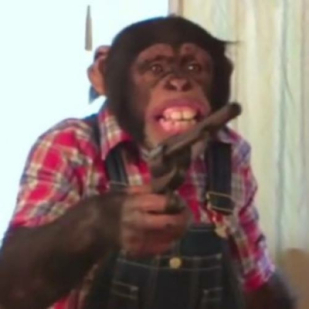
What do you think is going to happen when you run the code?
Will it bring back an Array of unique values?
It will without a doubt, but tell me, WHEN do you think that program of yours will finish execution?
You can test that out yourself, and I’ll see you in a week.
LINQ Supercharges Your Code
LINQ is very good at handling memory which is why its processing power is phenomenal.
I once ran a process that iterated through 25000 excel rows using a For Each Activity, and it took two hours.
Two frickin’ hours.
That was before I discovered LINQ, and when I tried the same process this time with LINQ, my jaw dropped.
That two hour process was reduced to less than a second.
I thought the system ran into some sort of error, because I didn’t believe it was possible for the system to process that much data in under a minute, let alone under a second.
But it happened.
And that is the moment when I accepted LINQ as my Lord and Saviour.
Now I spend my days reciting stories of his greatness, but it often falls on deaf ears since many prefer sinning on their smartphones, than working towards something worthwhile which would invite the Lord’s blessings.
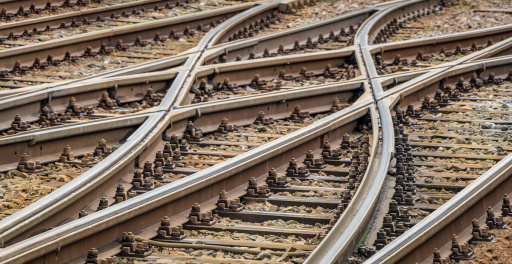
How do we iterate through a monstrous array in less time it would take me to say LINQ?
By using LIN-
ArrayVariable.Select(Function(s) s).Distinct.ToArray
The syntax might confuse you at first, but don’t worry. You will slowly get a hang of it.
Back To Our Monkey Business
Your manager walks in, takes a look at the output and says, “Not bad. This looks fine and all, but wouldn’t it look better if it was in order?” before walking back out to have another coffee.
Lucky for you, Microsoft has your back.
LINQ allows us to call “Methods” that do most, if not all, of the work for you.
We already explored them earlier. See if you can figure out where to use them next.
ArrayOfIntegers.OrderBy(Function(s) s).Distinct.ToArray
Confused?
I will say this again, none of what you read in your introduction will make sense to you, so stay calm.
You have to get comfortable with the syntax, and that happens through practice.
This is what I want you to do, head over to your UiPath Studio, open up a new process, create a random array and repeat whatever steps I have mentioned above.
If you run into any errors, then fix it yourself.
“You Are Supposed To Help Us!“
I won’t explain everything here.
I may explain few concepts here and there, but it’s up to you to cover every other aspect of it. You only retain what you invest effort into, so take it seriously and watch as your stock prices soar.
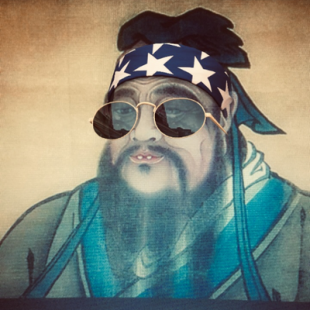
You can head over to YouTube and search for them cuz I ain’t tellin’ you shit.
-Confucius
I will also deliberately add errors into my LINQs that you will have to resolve yourself. This not only strengthens your ability to craft and debug your LINQs, but also abdicates me from any sort of responsibility to be right all the time, so I have killed two birds with one stone.
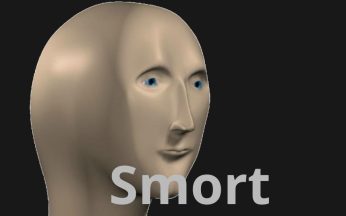
LINQ is not easy to learn, which is why learning it is worth the effort.
Heck, even I am still learning, but I figured it would be best to share whatever I have learned so far.
I will write more on LINQ and include proper screenshots from UiPath itself, so stay tuned.